异常处理
异常,就是不正常的意思。在生活中:医生说,你的身体某个部位有异常,该部位和正常相比有点不同,该部位的功能将受影响.在程序中的意思就是:
异常 :指的是程序在执行过程中,出现的非正常的情况,最终会导致JVM的非正常停止。
在Java等面向对象的编程语言中,异常本身是一个类,产生异常就是创建异常对象并抛出了一个异常对象。Java处理异常的方式是中断处理。
异常指的并不是语法错误,语法错了,编译不通过,不会产生字节码文件,根本不能运行.
Throwable
java.lang.Throwable
: 类似java语言中所有错误或异常的超类
异常就相当于程序得了一个小毛病(感冒,发烧),把异常处理掉,程序可以继续执行(吃点药,继续革命工作)
错误就相当于程序得了一个无法治愈的毛病(非典,艾滋),必须修改源代码,程序才能继续执行。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| package com.tipdm.Demo01;
import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date;
public class demo1 { public static void main(String[] args){ SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); Date date = null; try { date = sdf.parse("1999-09-09"); } catch (ParseException e) { e.printStackTrace(); } System.out.println(date);
int[] arr = {1, 2, 3}; try{ System.out.println(arr[3]); }catch (Exception e){ System.out.println(e); }
int[] arr1 = new int[1024*1024]; System.out.println("后续代码!"); } }
|
1 2 3 4 5
| Thu Sep 09 00:00:00 CST 1999 java.lang.ArrayIndexOutOfBoundsException: 3 后续代码!
进程已结束,退出代码0
|
异常的产生过程
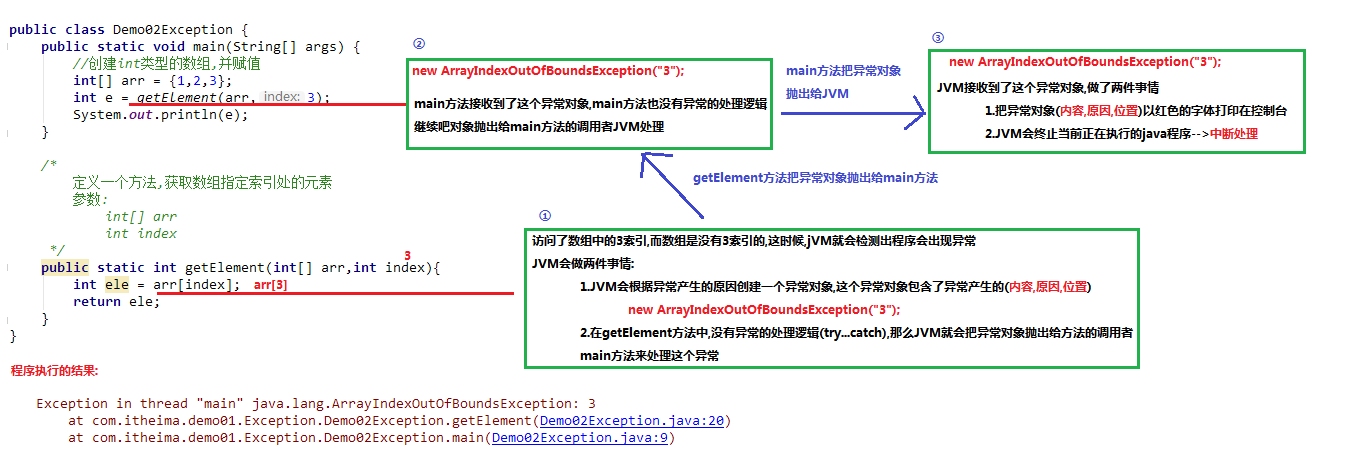
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.tipdm.Demo01;
public class demo2 { public static void main(String[] args) { int[] arr = {1, 2, 3}; int e = gerElement(arr, 3); System.out.println(e); }
public static int gerElement(int[] arr, int index){ int ele = arr[index]; return ele; } }
|
异常的处理
抛出异常throw
throw 关键字
作用:
- 可以使用throw关键字在指定的方法中抛出指定的异常
使用格式:
1
| throw new xxxException("异常产生的原因")
|
注意:
throw关键字必须写在方法的内部
throw关键字后边new的对象必须是Exception或者Exception的子类对象
throw关键字抛出指定的异常对象,我们就必须处理这个异常对象
throw关键字后边创建的是RuntimeException或者是 RuntimeException的子类对象,我们可以不处理,默认交给JVM处理(打印异常对象,中断程序)
throw关键字后边创建的是编译异常(写代码的时候报错),我们就必须处理这个异常,要么throws,要么try…catch…
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| package com.tipdm.Demo01;
import jdk.nashorn.internal.runtime.arrays.ArrayIndex;
public class demo3 { public static void main(String[] args) {
int[] array = new int[1]; gerElement(array, 1); }
public static int gerElement(int[] arr, int index){
if(arr == null){ throw new NullPointerException("传递的数组是空的!"); }
if(index < 0 || index > arr.length-1){ throw new ArrayIndexOutOfBoundsException("数组索引越界!"); } int ele = arr[index]; return ele; } }
|

Objects非空判断
1 2 3 4 5 6
| Objects非空判断 public static <T> T requireNonNull(T obj):查看指定引用对象不是null。 public static <T> T requireNonNull(T obj) { if (obj == null) throw new NullPointerException(); return obj;
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package com.tipdm.Demo01;
import java.util.Objects;
public class demo4 { public static void main(String[] args) { method(null); } public static void method(Object obj){
Objects.requireNonNull(obj, "传递的参数是null"); Objects.requireNonNull(obj); } }
|

throws声明异常
throws关键字:异常处理的第一种方式,交给别人处理
作用:
使用格式:
1 2 3 4 5
| 修饰符 返回值类型 方法名(参数列表) throws AAAException, BBBException, ...{ throw new AAAException("产生原因"); throw new BBBException("产生原因"); ... }
|
注意:
throws关键字必须写在方法声明处
throws关键字后边声明的异常必须是Exception或者是Exception的子类
方法内部如果抛出了多个异常对象,那么throws后边必须也声明多个异常
如果抛出的多个异常对象有子父类关系,那么直接声明父类异常即可
- 调用了一个声明抛出异常的方法,我们就必须的处理声明的异常要么继续使用throws声明抛出,交给方法的调用者处理,最终交给JVM要么try…catch…自己处理异常
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| package com.tipdm.Demo01;
import java.io.FileNotFoundException; import java.io.IOError; import java.io.IOException;
public class demo5 { public static void main(String[] args) throws IOException {
String str1 = "D:\\a.txt";
readFile(str1); }
public static void readFile(String fileName) throws IOException {
if(!fileName.endsWith(".txt")){ throw new IOException("文件的后缀名不对"); }
if(!fileName.equals("C:\\a.txt")){ throw new FileNotFoundException("传递的文件路径不是C:\\a.txt"); } System.out.println("路径没有问题,读取文件!"); } }
|

try…catch…处理异常
try…catch… 异常处理的第二种方式,自己处理异常
格式:
1 2 3 4 5 6 7 8 9 10
| try{ 可能产生异常的代码 }catch(Exception e){ 异常的处理逻辑,产生异常对象之后,怎么处理异常对象 一般会把工作中,会把异常的信息记录到一个日志中 } catch(异常类名 变量名){ ... } ...
|
注意:
try中可能抛出多个异常对象,那么就可以使用多个catch来处理这些异常对象
如果try中产生了异常,那么就会执行catch中的异常处理逻辑,执行完毕catch中的处理逻辑,继续执行try… catch之后的代码;如果try中没有产生异常,那么就不会执行catch中异常的处理逻辑,执行try中的代码,继续执行try…catch…之后的代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| package com.tipdm.Demo01;
import java.io.FileNotFoundException; import java.io.IOException;
public class demo6 { public static void main(String[] args) { try { readFile("C:\\a.tx"); System.out.println("资源释放!"); } catch (IOException e) {
e.printStackTrace(); }finally { System.out.println("资源释放"); } System.out.println("后续代码"); }
public static void readFile(String fileName) throws IOException { if(!fileName.endsWith(".txt")){ throw new IOException("文件的后缀名不对"); } System.out.println("路径没有问题,读取文件!"); } }
|
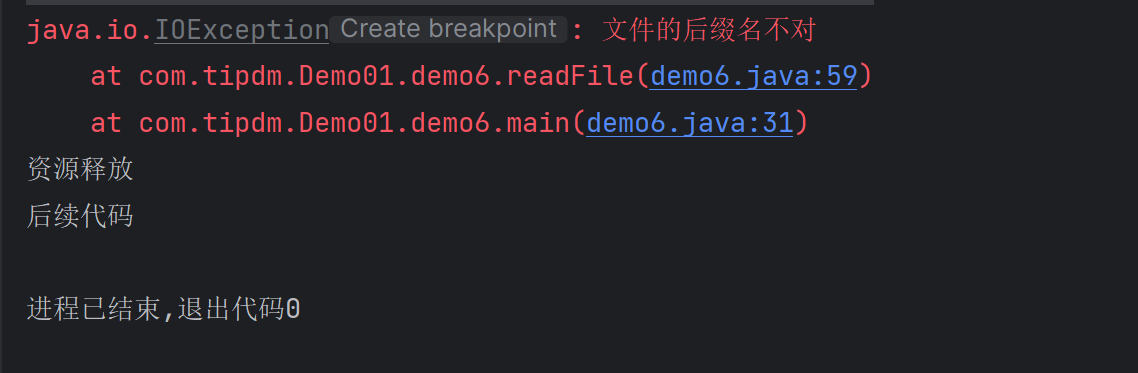
finally代码块
finally 代码块
格式:
1 2 3 4 5 6 7 8 9 10 11
| try{ 可能产生异常的代码 }catch(Exception e){ 异常的处理逻辑,产生异常对象之后,怎么处理异常对象 一般会把工作中,会把异常的信息记录到一个日志中 } ... catch(异常类名 变量名){ }finally{ 无论是否出现异常都会执行 }
|
注意:
finally不能单独使用,必须和try一起使用
finally一般用于资源释放(资源回收),无论程序是否出现异常,最后都要资源释放(IO流)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| package com.tipdm.Demo01;
import java.io.IOException;
public class demo7 { public static void main(String[] args) { try { readFile("C:\\a.tt"); System.out.println("资源释放111111"); } catch (IOException e) { e.printStackTrace(); }finally { System.out.println("资源释放2222222"); } }
public static void readFile(String fileName) throws IOException { if(!fileName.endsWith(".txt")){ throw new IOException("文件的后缀名不对"); } System.out.println("路径没有问题,读取文件!"); } }
|
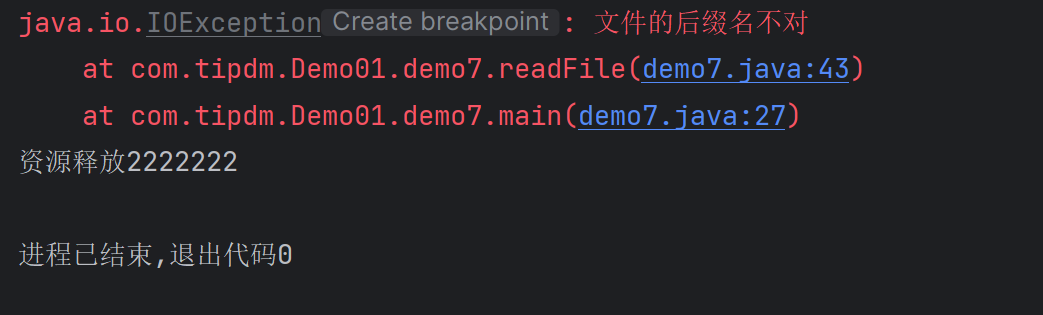
异常的注意事项
多个异常使用捕获又该如何处理呢?
- 多个异常分别处理。
- 多个异常一次捕获,多次处理。
- 多个异常一次捕获一次处理。
一般我们是使用一次捕获多次处理方式,格式如下:
1 2 3 4 5 6 7 8 9
| try{ 编写可能会出现异常的代码 }catch(异常类型A e){ 当try中出现A类型异常,就用该catch来捕获. 处理异常的代码 }catch(异常类型B e){ 当try中出现B类型异常,就用该catch来捕获. 处理异常的代码 }
|
注意:这种异常处理方式,要求多个catch中的异常不能相同,并且若catch中的多个异常之间有子父类异常的关系,那么子类异常要求在上面的catch处理,父类异常在下面的catch处理。
运行时异常被抛出可以不处理。即不捕获也不声明抛出。
如果finally有return语句,永远返回finally中的结果,避免该情况.
如果父类抛出了多个异常,子类重写父类方法时,抛出和父类相同的异常或者是父类异常的子类或者不抛出异常。
父类方法没有抛出异常,子类重写父类该方法时也不可抛出异常。此时子类产生该异常,只能捕获处理,不能声明抛出
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75
| package com.tipdm.Demo01;
import java.util.ArrayList;
public class demo8 { public static void main(String[] args) {
int[] arr = {1, 2, 3}; ArrayList<Integer> list = new ArrayList<>(); list.add(1); list.add(2); list.add(3);
try{ System.out.println(arr[3]); }catch (ArrayIndexOutOfBoundsException e){ System.out.println(e); } try{ System.out.println(list.get(3)); }catch (IndexOutOfBoundsException e){ System.out.println(e); } System.out.println("==========================");
try{ System.out.println(arr[3]); System.out.println(list.get(3)); }catch (ArrayIndexOutOfBoundsException e){ System.out.println(e); }catch (IndexOutOfBoundsException e){ System.out.println(e); }
System.out.println("=====================");
try{ System.out.println(arr[3]); System.out.println(list.get(3)); }catch (Exception e){ System.out.println(e); }
System.out.println(arr[3]); System.out.println(list.get(3)); System.out.println("后续代码!!!!!!!!!!!"); } }
|
如果finally有return语句,永远返回finally中的结果,避免该情况.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.tipdm.Demo01;
public class demo9 { public static void main(String[] args) { int i = getA(); System.out.println(i); }
public static int getA(){ int a = 10; try{ return a; }catch (Exception e){ System.out.println(e); }finally { a = 100; return a; } } }
|
子父类的异常:
如果父类抛出了多个异常,子类重写父类方法时,抛出和父类相同的异常或者是父类异常的子类或者不抛出异常。父类方法没有抛出异常,子类重写父类该方法时也不可抛出异常。此时子类产生该异常,只能捕获处理,不能声明抛出.
注意:父类异常是什么样,子类异常就什么样
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| package com.tipdm.Demo01;
public class Fu { public void show01() throws NullPointerException, ClassCastException{} public void show02() throws IndexOutOfBoundsException{} public void show03() throws IndexOutOfBoundsException{} public void show04() {} }
class Zi extends Fu{ public void show01() throws NullPointerException, ClassCastException{} public void show02() throws ArrayIndexOutOfBoundsException{} public void show03() {}
public void show04() { try{ throw new Exception("编译器异常"); }catch (Exception e){ e.printStackTrace(); } } }
|
自定义异常类
自定义异常类:
java提供的异常类,不够我们使用,需要自己定义一些异常类
格式:
1 2 3 4
| public class XXXException extends Exception | RuntimeException{ 添加一个空参数的构造方法 添加一个带异常信息的构造方法 }
|
注意:
自定义异常类一般都是以Exception结尾,说明该类是一个异常类
自定义异常类,必须继承Exception或者RuntimeException
继承 Exception :编译异常
继承RuntimeException :运行异常
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| package com.tipdm.Demo02;
public class RegisterException extends Exception {
public RegisterException() { }
public RegisterException(String message) { super(message); }
}
|
要求:我们模拟注册操作,如果用户名已存在,则抛出异常并提示:亲,该用户名已经被注册。(throws声明)
分析:
使用数组保存已经注册过的用户名(数据库)
使用Scanner获取用户输入的注册的用户名(前端,页面)
定义一个方法,对用户输入的注册的用户名进行判断
遍历存储已经注册过用户名的数组,获取每个用户名
使用获取到的用户名和用户输入的用户名进行比较
true:
用户名已经存在,抛出RegisterException异常,告知用户”该用户名已经被注册”;
false:
继续遍历比较
如果循环结束了,还没有找到重复的用户名,提示用户”恭喜您,注册成功!”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package com.tipdm.Demo02;
import java.util.Scanner;
public class RegisterTest { static String[] usernames = {"张三", "李四", "王五"};
public static void main(String[] args) throws RegisterException { System.out.print("请输入用户名:"); Scanner sc = new Scanner(System.in); String username = sc.next(); check(username); }
public static void check(String username) throws RegisterException { for (String name : usernames) { if(name.equals(username)){ throw new RegisterException("该用户名已经被注册"); } } System.out.println("恭喜您,注册成功!"); } }
|
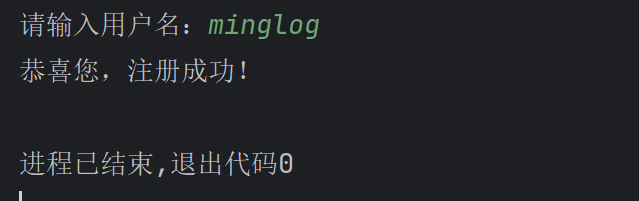

要求:我们模拟注册操作,如果用户名已存在,则抛出异常并提示:亲,该用户名已经被注册。(try…catch…处理)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| package com.tipdm.Demo02;
import java.util.Scanner;
public class RegisterTest1 { static String[] usernames = {"张三", "李四", "王五"};
public static void main(String[] args){ System.out.print("请输入用户名:"); Scanner sc = new Scanner(System.in); String username = sc.next(); check(username); }
public static void check(String username){ for (String name : usernames) { if(name.equals(username)){ try { throw new RegisterException("该用户名已经被注册"); } catch (RegisterException e) { e.printStackTrace(); return; } } } System.out.println("恭喜您,注册成功!"); } }
|
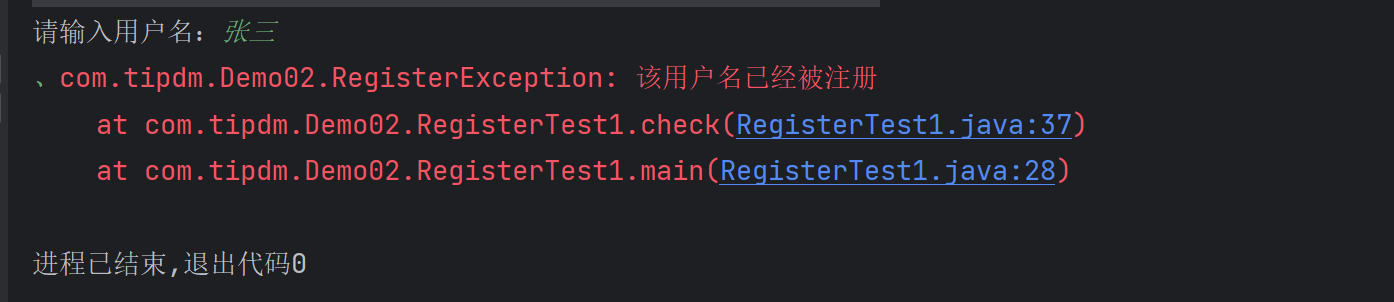