datetime库
date, datetime, time 和 timezone 通用特征属性
- 这些类型的对象都是不可变的。
- 这些类型的对象都是可哈希的,这意味着它们可被作为字典的键。
- 这些类型的对象支持通过
pickle
模块进行高效的封存。
库结构:
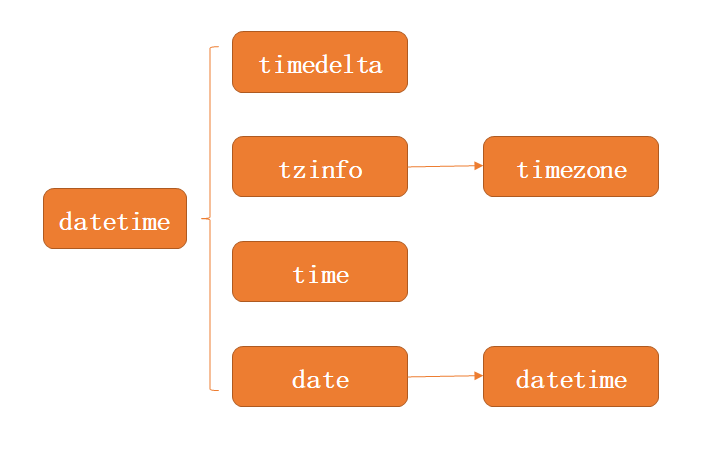
timedelta对象
timedalta对象表示两个date或者time的时间间隔
timedelta定义
为了保证表达方式的唯一性,输出结果会以days, seconds, microseconds标准格式处理。
1 2 3 4 5 6 7 8
| delta = dtm.timedelta( days=50, seconds=27, microseconds=10, minutes=5, weeks=2 ) delta
|
datetime.timedelta(days=64, seconds=327, microseconds=10)
1 2
| d1 = dtm.timedelta(minutes=2) d1
|
datetime.timedelta(seconds=120)
1 2
| d2 = dtm.timedelta(seconds=30) d2
|
datetime.timedelta(seconds=30)
属性
1 2 3
| print(d1.days) print(d1.seconds) print(d1.microseconds)
|
0
120
0
方法
1
| (d1 + d2).total_seconds()
|
150.0
运算
与标量间进行运算
datetime.timedelta(seconds=240)
datetime.timedelta(seconds=60)
datetime.timedelta(seconds=17, microseconds=142857)
两个timedelta进行运算
datetime.timedelta(seconds=150)
datetime.timedelta(seconds=90)
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-47-109a12497ebf> in <module>
1 # 无法做乘法运算
----> 2 d1 * d2
TypeError: unsupported operand type(s) for *: 'datetime.timedelta' and 'datetime.timedelta'
1.3333333333333333
1
datetime.timedelta(seconds=30)
datetime.timedelta(days=-1, seconds=86280)
datetime.timedelta(seconds=90)
'-1 day, 23:58:30'
'datetime.timedelta(seconds=120)'
比较
True
False
False
True
tzinfo对象
这是一个抽象类,也就是说该类不应被直接实例化。在使用时,一般定义tzinfo的子类来捕获有关特定时区的信息。
将本地时间与 UTC 时差返回为一个 timedelta 对象,如果本地时区在 UTC 以东则为正值。 如果本地时区在 UTC 以西则为负值。
将夏令时(DST)调整返回为一个 timedelta 对象,如果 DST 信息未知则返回 None
将对应于 datetime 对象 dt 的时区名称作为字符串返回。
此方法会由默认的 datetime.astimezone() 实现来调用。 当被其调用时,dt.tzinfo 为 self,并且 dt 的日期和时间数据会被视为表示 UTC 时间,fromutc() 的目标是调整日期和时间数据,返回一个等价的 datetime 来表示 self 的本地时间.
timezone对象
class datetime.timezone(offset, name=None)
- offset 参数必须指定为一个 timedelta 对象,表示本地时间与 UTC 的时差。 它必须严格限制于 -timedelta(hours=24) 和 timedelta(hours=24) 之间,否则会引发 ValueError。
- name 参数是可选的。 如果指定则必须为一个字符串,它将被用作 datetime.tzname() 方法的返回值。
timezone 类是 tzinfo 的子类,它的每个实例都代表一个以与 UTC 的固定时差来定义的时区。
1 2
| td = dtm.timedelta(hours=8)
|
1 2 3
| tz1 = dtm.timezone(td, '新时区') tz1
|
datetime.timezone(datetime.timedelta(seconds=28800), '新时区')
1 2
| datetime33 = dtm.datetime(2022, 11, 22, 16, 4, 22, tzinfo=tz1)
|
1 2
| tz1.utcoffset(datetime33)
|
datetime.timedelta(seconds=28800)
'新时区'
1 2
| tz1.fromutc(datetime33)
|
datetime.datetime(2022, 11, 23, 0, 4, 22, tzinfo=datetime.timezone(datetime.timedelta(seconds=28800), '新时区'))
date对象
class datetime.date(year, month, day)
date
对象代表一个理想化中的日期(年、月和日)
类方法
datetime.date(2022, 11, 22)
1 2
| dtm.date.fromtimestamp(time.time())
|
datetime.date(2022, 11, 22)
1 2
| dtm.date.fromisoformat('2022-11-21')
|
datetime.date(2022, 11, 21)
1 2 3
|
dtm.date.fromisocalendar(2022, 11, 2)
|
datetime.date(2022, 3, 15)
1 2
| dtm.date.fromordinal(738481)
|
datetime.date(2022, 11, 22)
类属性
datetime.date(1, 1, 1)
datetime.date(9999, 12, 31)
datetime.timedelta(days=1)
实例属性
1
| date1 = dtm.date.today()
|
1
| date2 = dtm.date(2022, 12, 31)
|
1 2 3
| print(date1.year) print(date1.month) print(date1.day)
|
2022
11
22
运算
1 2
| d3 = dtm.timedelta(days=10) d3
|
datetime.timedelta(days=10)
datetime.date(2022, 11, 22)
datetime.date(2022, 12, 2)
datetime.date(2022, 11, 12)
datetime.timedelta(days=39)
比较
True
实例方法
1 2 3 4
| print(date2) date2 = date2.replace(year=2021, day=30) print(date2)
|
2022-12-31
2021-12-30
time.struct_time(tm_year=2022, tm_mon=11, tm_mday=22, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=1, tm_yday=326, tm_isdst=-1)
1 2 3 4 5
| print(date1.toordinal())
print((dtm.date.today() - dtm.date(1, 1, 1) + dtm.timedelta(days=1)).days)
|
738481
738481
1 2
| date1.fromordinal(date1.toordinal())
|
datetime.date(2022, 11, 22)
1
2
1 2 3
| dtm.date.today().isocalendar()
|
(2022, 47, 2)
'2022-11-22'
'Tue Nov 22 00:00:00 2022'
1 2
| date1.strftime('%a %b %d %H-%M-%S %Y')
|
'Tue Nov 22 00-00-00 2022'
time对象
class datetime.time(hour=0, minute=0, second=0, microsecond=0, tzinfo=None, *, fold=0)
一个 time 对象代表某日的(本地)时间,它独立于任何特定日期,并可通过 tzinfo 对象来调整。
类属性
datetime.time(0, 0)
datetime.time(23, 59, 59, 999999)
datetime.timedelta(microseconds=1)
实例属性
1 2 3
| t1 = dtm.time(16, 17, 22, 222333) print(t1)
|
16:17:22.222333
1 2 3
| t2 = dtm.time(16, 17, 22, 222333, tzinfo=tz1) t2
|
datetime.time(16, 17, 22, 222333, tzinfo=datetime.timezone(datetime.timedelta(seconds=28800), '新时区'))
1 2 3 4 5 6
| print(t2.hour) print(t2.minute) print(t2.second) print(t2.microsecond) print(t2.tzinfo)
|
16
17
22
222333
新时区
1 2
| dtm.time.fromisoformat('17:09:11.333222+07:00')
|
datetime.time(17, 9, 11, 333222, tzinfo=datetime.timezone(datetime.timedelta(seconds=25200)))
'16:17:22.222333'
1 2
| t1.strftime('%H %M %S')
|
'16 17 22'
1 2 3 4
| print('修改前:', t1) t1 = t1.replace(tzinfo=tz1) print('修改后:', t1)
|
修改前: 16:17:22.222333
修改后: 16:17:22.222333+08:00
datetime.timedelta(seconds=28800)
'新时区'
datetime对象
class datetime.datetime(year, month, day, hour=0, minute=0, second=0, microsecond=0, tzinfo=None, *, fold=0)
datetime对象是包含来自date对象和time对象的所有信息的单一对象。
类方法
datetime.datetime(2022, 11, 22, 17, 36, 45, 863696)
datetime.datetime(2022, 11, 22, 9, 36, 46, 103993)
1 2 3
| dtm.datetime.now(tz=tz1)
|
datetime.datetime(2022, 11, 22, 17, 36, 46, 376150, tzinfo=datetime.timezone(datetime.timedelta(seconds=28800), '新时区'))
datetime.datetime(2022, 11, 22, 9, 36, 46, 520149)
datetime.datetime(2022, 11, 22, 17, 36, 47, 247660, tzinfo=datetime.timezone(datetime.timedelta(seconds=28800), '新时区'))
1 2
| dtm.datetime.fromtimestamp(time.time())
|
datetime.datetime(2022, 11, 22, 17, 36, 47, 665319)
1 2
| dtm.datetime.utcfromtimestamp(time.time())
|
datetime.datetime(2022, 11, 22, 9, 36, 48, 113233)
1 2
| dtm.datetime.fromordinal(738481)
|
datetime.datetime(2022, 11, 22, 0, 0)
1 2 3
|
dtm.datetime.fromisoformat('2022-11-21 14:27:22.222333')
|
datetime.datetime(2022, 11, 21, 14, 27, 22, 222333)
1 2 3
| print(dtm.datetime.isocalendar(dtm.datetime.now())) print(dtm.datetime.fromisocalendar(2022, 47, 2))
|
(2022, 47, 2)
2022-11-22 00:00:00
1 2
| dtm.datetime.strptime('Tue Nov 22 14:36:25 2022', '%a %b %d %H:%M:%S %Y')
|
datetime.datetime(2022, 11, 22, 14, 36, 25)
1 2
| dtm.datetime.combine(date1, t1)
|
datetime.datetime(2022, 11, 22, 16, 17, 22, 222333, tzinfo=datetime.timezone(datetime.timedelta(seconds=28800), '新时区'))
类属性
datetime.datetime(1, 1, 1, 0, 0)
datetime.datetime(9999, 12, 31, 23, 59, 59, 999999)
datetime.timedelta(microseconds=1)
实例属性
1 2
| datetime1 = dtm.datetime(2022, 11, 21, 14, 22, 36, 666555) datetime1
|
datetime.datetime(2022, 11, 21, 14, 22, 36, 666555)
1 2
| datetime2 = dtm.datetime.now() datetime2
|
datetime.datetime(2022, 11, 22, 17, 38, 45, 292189)
1 2 3 4 5 6 7 8
| print(datetime1.year) print(datetime1.month) print(datetime1.hour) print(datetime1.minute) print(datetime1.second) print(datetime1.microsecond) print(datetime1.tzinfo)
|
2022
11
14
22
36
666555
None
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
<ipython-input-197-c098b83ee9f7> in <module>
----> 1 datetime1.year = 2022 # 实例属性不可更改
AttributeError: attribute 'year' of 'datetime.date' objects is not writable
运算
datetime.timedelta(days=1, seconds=11768, microseconds=625634)
1 2 3 4
| d1 = dtm.timedelta(days=1)
datetime1 - d1
|
datetime.datetime(2022, 11, 20, 14, 22, 36, 666555)
比较
True
实例方法
datetime.date(2022, 11, 21)
datetime.time(14, 22, 36, 666555)
datetime.time(14, 22, 36, 666555)
1 2 3 4
| print('修改前:', datetime1) datetime1 = datetime1.replace(year=2025) print('修改后:', datetime1)
|
修改前: 2022-11-21 14:22:36.666555
修改后: 2025-11-21 14:22:36.666555
1 2
| datetime1 = datetime1.astimezone(tz=tz1)
|
'新时区'
datetime.timedelta(seconds=28800)
'新时区'
time.struct_time(tm_year=2025, tm_mon=11, tm_mday=21, tm_hour=14, tm_min=22, tm_sec=36, tm_wday=4, tm_yday=325, tm_isdst=-1)
1 2
| datetime1.utctimetuple()
|
time.struct_time(tm_year=2025, tm_mon=11, tm_mday=21, tm_hour=6, tm_min=22, tm_sec=36, tm_wday=4, tm_yday=325, tm_isdst=0)
pandas
Timestamp对象
构造方法
利用字符串格式时间数据转化
pandas支持智能的将各种格式的时间数据转化为标准时间戳格式
1 2 3
| tm1 = pd.Timestamp.now() tm1
|
Timestamp('2022-11-22 18:04:34.850491')
1 2
| tm2 = pd.Timestamp('2022-11-21 16:12:12') tm2
|
Timestamp('2022-11-21 16:12:12')
1 2
| tm3 = pd.Timestamp('2022/11/21 16:12:12') tm3
|
Timestamp('2022-11-21 16:12:12')
1 2 3
| tm4 = pd.to_datetime(time.ctime()) tm4
|
Timestamp('2022-11-22 18:04:59')
利用时间戳进行转换
1
| pd.Timestamp(1669112020.1238306, unit='s')
|
Timestamp('2022-11-22 10:13:40.123830557')
1 2
| d = pd.Timestamp(1669112020.1238306, unit='s', tz=tz1) d
|
Timestamp('2022-11-22 18:13:40.123830557+0800', tz='新时区')
通过直接指定相应时间属性值
1 2
| pd.Timestamp(year=2022, month=11, day=21, hour=20, minute=28, second=30, microsecond=30)
|
Timestamp('2022-11-21 20:28:30.000030')
1 2
| d2 = pd.Timestamp(2022, 11, 30, 20, 26,30) d2
|
Timestamp('2022-11-30 20:26:30')
属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| print(d.tz) print(d.tzinfo)
print(d.asm8)
print(d.weekofyear)
print(d.day_of_week)
print(d.day_of_year)
print(d.days_in_month) print(d.daysinmonth)
print(d.year) print(d.month) print(d.day) print(d.hour) print(d.minute) print(d.second) print(d.nanosecond) print(d.microsecond) print(d.quarter)
print(d.is_leap_year) print(d2.is_month_end) print(d.is_month_start) print(d.is_year_end) print(d.is_year_start)
|
新时区
新时区
2022-11-22T10:13:40.123830557
47
1
326
30
30
2022
11
22
18
13
40
557
123830
4
False
True
False
False
False
方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| print(d.ctime())
print(d.day_name())
print(d.timestamp())
print(d.fromtimestamp(d.timestamp()))
print(d.utcfromtimestamp(d.timestamp()))
print(d.toordinal() )
print(d.fromordinal(d.toordinal()))
print(d.isocalendar())
print(d.timetuple())
print(d.tzname())
print(d.isoformat())
|
Tue Nov 22 18:13:40 2022
Tuesday
1669112020.123831
2022-11-22 18:13:40.123831
2022-11-22 10:13:40.123831
738481
2022-11-22 00:00:00
(2022, 47, 2)
time.struct_time(tm_year=2022, tm_mon=11, tm_mday=22, tm_hour=18, tm_min=13, tm_sec=40, tm_wday=1, tm_yday=326, tm_isdst=-1)
新时区
2022-11-22T18:13:40.123830557+08:00
Timedelta时间段
构造方法
通过字符串格式进行定义
1 2
| pd.Timedelta('2days 2hours 15minutes 30seconds')
|
Timedelta('2 days 02:15:30')
1 2
| pd.Timedelta('2d2h15min30s')
|
Timedelta('2 days 02:15:30')
通过整数值与指定单位定义
1
| pd.Timedelta(150, unit='s')
|
Timedelta('0 days 00:02:30')
1
| pd.Timedelta(1.5, unit='d')
|
Timedelta('1 days 12:00:00')
直接指定相应属性定义
1
| pd.Timedelta(days=2, hours=15, minutes=30, seconds=30)
|
Timedelta('2 days 15:30:30')
1 2 3 4
| print(pd.to_timedelta('2d')) print(pd.to_timedelta('2d3m')) print(pd.to_timedelta(200, unit='s'))
|
2 days 00:00:00
2 days 00:03:00
0 days 00:03:20
date_range时间区间
其中每个元素都为Timestamp对象
指定起点、终点和数据量
1
| pd.date_range(start='2022-11-1', end='2022-11-30', periods=30)
|
DatetimeIndex(['2022-11-01', '2022-11-02', '2022-11-03', '2022-11-04',
'2022-11-05', '2022-11-06', '2022-11-07', '2022-11-08',
'2022-11-09', '2022-11-10', '2022-11-11', '2022-11-12',
'2022-11-13', '2022-11-14', '2022-11-15', '2022-11-16',
'2022-11-17', '2022-11-18', '2022-11-19', '2022-11-20',
'2022-11-21', '2022-11-22', '2022-11-23', '2022-11-24',
'2022-11-25', '2022-11-26', '2022-11-27', '2022-11-28',
'2022-11-29', '2022-11-30'],
dtype='datetime64[ns]', freq=None)
指定起点、终点和步长
1
| pd.date_range(start='2022-11-1', end='2022-11-30', freq='3d')
|
DatetimeIndex(['2022-11-01', '2022-11-04', '2022-11-07', '2022-11-10',
'2022-11-13', '2022-11-16', '2022-11-19', '2022-11-22',
'2022-11-25', '2022-11-28'],
dtype='datetime64[ns]', freq='3D')
指定起点、数据量和步长
1
| pd.date_range(start='2022-11-3', periods=10, freq='2d')
|
DatetimeIndex(['2022-11-03', '2022-11-05', '2022-11-07', '2022-11-09',
'2022-11-11', '2022-11-13', '2022-11-15', '2022-11-17',
'2022-11-19', '2022-11-21'],
dtype='datetime64[ns]', freq='2D')
指定终点、数据量和步长
1
| pd.date_range(end='2022-11-3 9:00:00', periods=10, freq='3d')
|
DatetimeIndex(['2022-10-07 09:00:00', '2022-10-10 09:00:00',
'2022-10-13 09:00:00', '2022-10-16 09:00:00',
'2022-10-19 09:00:00', '2022-10-22 09:00:00',
'2022-10-25 09:00:00', '2022-10-28 09:00:00',
'2022-10-31 09:00:00', '2022-11-03 09:00:00'],
dtype='datetime64[ns]', freq='3D')